题目描述
JAVA 解法
class Solution {
public int myAtoi(String s) {
// 将传进来的字符串转换为字符数组
char[] chars = s.toCharArray();
// 获取字符数组的长度
int n = chars.length;
// 定义全局索引起始位置
int idx = 0;
while (idx < n && chars[idx] == ' ') {
// 去掉空格
idx++;
}
if (idx == n) {
//去掉所有空格后若到了末尾则停止程序
return 0;
}
// 标记是否为负
boolean negative = false;
if (chars[idx] == '-') {
//遇到负号,则标记为负
negative = true;
// 继续下一个
idx++;
} else if (chars[idx] == '+') {
// 遇到正号,则直接下一个
idx++;
} else if (!Character.isDigit(chars[idx])) {
// 若第一个就遇到非数字非正负符号的其他字符则停止程序
return 0;
}
// 定义一个存储最终结果的变量
int ans = 0;
// 数组下标不越界且字符为数字时进行遍历
while (idx < n && Character.isDigit(chars[idx])) {
// 由于字符 '0' 到 '9' 的 ASCII 值连续,通过字符的 ASCII 值作差即可巧妙转换为字符对应的整数值
int digit = chars[idx] - '0';
// 每一次循环都要防止数值过大导致溢出,要判断 ans * 10 + digit 是否大于 Integer.MAX_VALUE,但是直接 ans * 10 + digit 的话可能在此直接溢出,所以为了避免运算时溢出,将等式移位,将乘变成除
if (ans > (Integer.MAX_VALUE - digit) / 10) {
// 如果上面判断该数为负数的话,返回 Integer.MIN_VALUE,为正的话返回,Integer.MAX_VALUE
return negative? Integer.MIN_VALUE : Integer.MAX_VALUE;
}
// 由于遍历是从左向右遍历的,因此只需要每次用 ans 乘以 10 并加上当前的值即可还原数对应的值
ans = ans * 10 + digit;
// 下一个
idx++;
}
// 返回最终结果,若为正则返回正数,若为负则返回负数
return negative? -ans : ans;
}
}
题解分析
感谢您的来访,获取更多精彩文章请收藏本站。
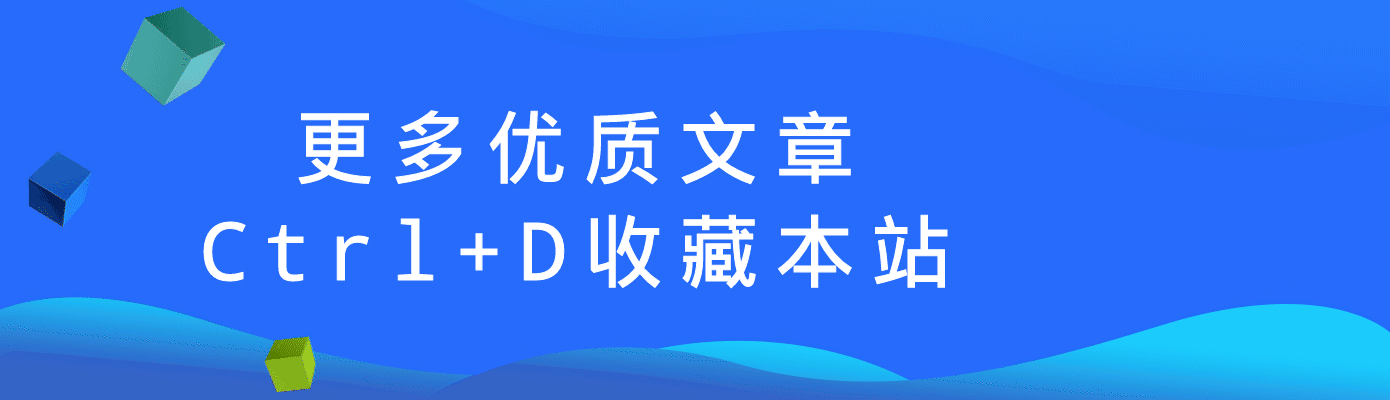
© 版权声明
THE END