Problem Description
Given a linked list, return the node where the cycle begins. If there is no cycle, return null
.
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next
pointer. Internally, pos
is used to denote the index of the node that tail’s next
pointer is connected to (0-indexed). It is -1
if there is no cycle. Note that pos
is not passed as a parameter.
Do not modify the linked list.
Approach
To detect the starting node of a cycle in a linked list, we can use the Floyd’s cycle-finding algorithm. This algorithm involves using two pointers, fast
and slow
, initially pointing to the head of the linked list.
- Initialize the
fast
andslow
pointers to the head of the linked list. - Move the
fast
pointer by two steps and theslow
pointer by one step at each iteration. - If there is a cycle in the linked list, the
fast
andslow
pointers will eventually meet at the same node inside the cycle. - Once the
fast
andslow
pointers meet, we reset one of the pointers (e.g.,slow
) to the head of the linked list. - Then, we move both the
fast
andslow
pointers one step at a time until they meet again. - The node where they meet again is the starting point of the cycle.
Implementation with Comments
public ListNode detectCycle(ListNode head) {
// Initialize fast and slow pointers
ListNode fast = head;
ListNode slow = head;
// Move the fast and slow pointers until they meet
while (fast != null && fast.next != null) {
slow = slow.next;
fast = fast.next.next;
// Check if the pointers have met inside the cycle
if (fast == slow) {
// Reset one pointer to the head and move both pointers one step at a time until they meet again
ListNode index1 = slow;
ListNode index2 = head;
while (index1 != index2) {
index1 = index1.next;
index2 = index2.next;
}
// Return the starting node of the cycle
return index1;
}
}
// If no cycle is found, return null
return null;
}
Complexity Analysis
- Time complexity: O(N), where N is the number of nodes in the linked list. We need to traverse the linked list at most twice: once to detect the cycle and once to find the starting node of the cycle.
- Space complexity: O(1), using only constant extra space.
The Floyd’s cycle-finding algorithm allows us to detect the starting node of a cycle in a linked list in an efficient manner. By manipulating the pointers correctly, we can identify the cycle and determine its starting node without using additional space beyond the original linked list.
Understanding this algorithm is crucial for solving related problems that involve detecting cycles in linked lists.
感谢您的来访,获取更多精彩文章请收藏本站。
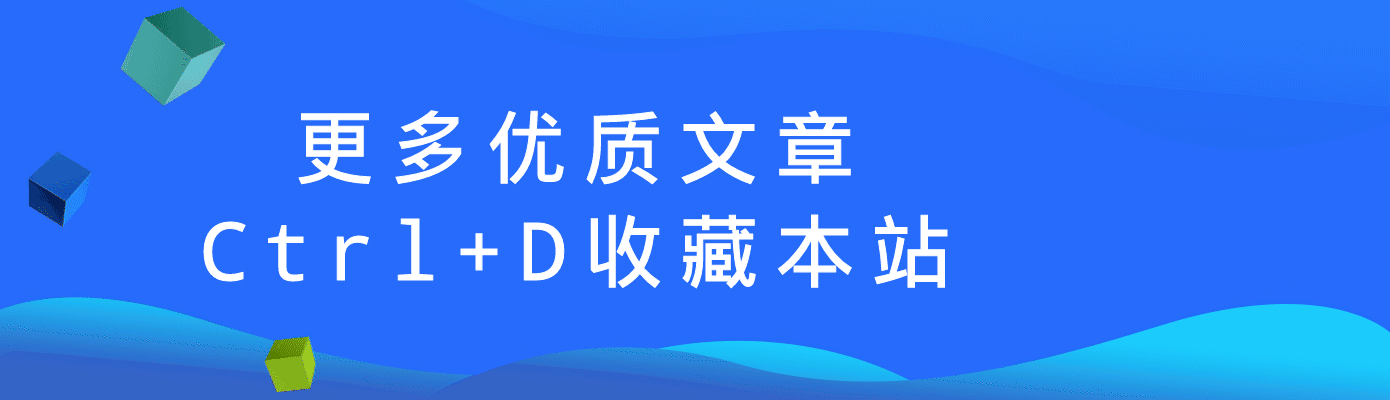